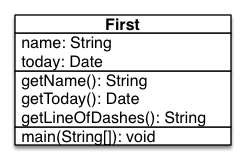
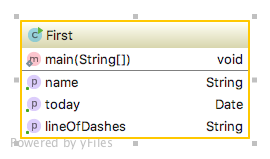
ICT-4361 Homework 1
Purpose
This exercise is to familiarize the student
with the programming tools for the course, and to give the student an
early opportunity to receive exercise feedback from the instructor.
Additionally, the student will have some
experience accessing Java resources over the Internet.
Please address this problem as early as
possible to ensure you have a proper environment available for
accomplishing all other homework assignments.
What to Hand In
For this, as well as future programming
exercises, hand in a listing of the program (which must be formatted
in a reasonable style), and sample run(s) of the program.
Please pay attention to your code indentation to ensure your paper is easy to read
and understand.
Canvas submission instructions:
Please combine multiple files into a single "zip" archive, and save it in a location
that you will remember. When you are ready to submit the assignment, open the assignment
you are submitting a soluction for, and attach your file.
Problems
- A Compiler to Know And Love
-
Ensure you have a Java development environment installed and working.
Please ensure you have an up-to-date version of the Java compiler and your selected development environment.
- Netbeans: www.netbeans.org;
- Eclipse: www.eclipse.org;
- Oracle: www.oracle.com/us/technologies/java/.
See the instructor handouts beginning with a "2" for details on specific environments (including the command line environment) for how to create, compile, and run Java programs. While these might not all be up to the latest version, the steps to create and run a program are the same.
-
Enter the following simple program into the file First.java
// ICT 4361 // Author: Put Your Name Here // Filename: First.java import java.util.Date; public class First { private Date today = new Date(); private String name = "Put Your Name Here"; public String getName() { return name; } public Date getToday() { return today; } public String getLineOfDashes() { return "----------------------------------------------------------------"; } public static void main (String[] args) { First theFirst = new First(); System.out.println(theFirst.getLineOfDashes()); System.out.println("Today is " + theFirst.getToday()); System.out.println(theFirst.getLineOfDashes()); System.out.println("My name is " + theFirst.getName()); System.out.println(theFirst.getLineOfDashes()); System.out.println("I've just finished my first Java homework!"); } }
-
Replace your own name for all the "Put Your Name Here" placeholders, compile it, and run it. Capture the results for output.
-
- A Tutorial To Know and Love
-
This assignment is to familiarize you with some of the many online resources for Java. Sun has a tutorial on its site for learning the Java language (docs.oracle.com/javase/tutorial/index.html) It's rather terse, but is example driven. The Java site also has a number of other helpful resources for the course, including on-line APIs, forums for discussion of the use of Java features, and other useful content. Visit the site and bookmark it. Read through the "Strings" section, and try the examples (not to hand in, unless you have a question). You may also find it helpful as a supplement to the course handouts.
-
- Read the syllabus for the course, and include a written sentence either as a comment in your code for the first problem, or as a separate text file (.txt) that indicates you have read and understand the syllabus for this course.
Notes
-
Much of Java is learned through on-line resources. Becoming familiar with these is a part of the language. -
The First.java program defines a single class, named First. -
The class is public so that instances of it may be created. -
The class has two private data members, called name and today -
The private data members may not be referenced without creating an object of the class First. Further, objects of other classes cannot reference the private data members of First at all. -
The main program is marked as public, so it may be accessed -
The main program is marked as static, so that method may be accessed without a First instance. -
The main program can create an instance of First, and use its public methods, getName(), getToday(), and getLineOfDashes() to obtain information from its created instance of First
Evaluation
Criteria | Weight |
---|---|
Problem I: Changed the Author in the top comment on line 2 | 15% |
Problem I: Changed the name in the private String initialization on line 6 | 25% |
Problem I: Compiled program, ran program, captured output | 45% |
Problem III: Acknowledged reading the syllabus | 15% |