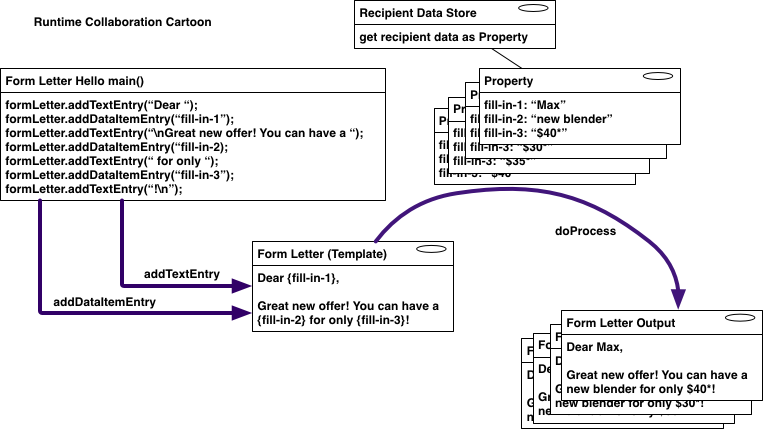
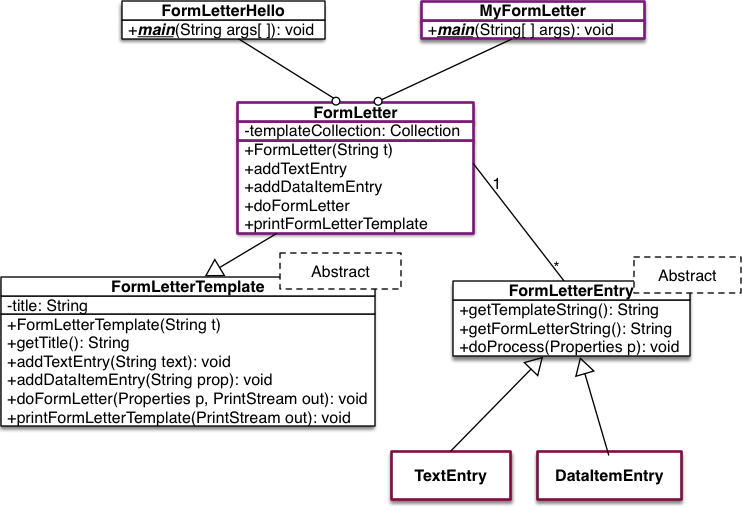
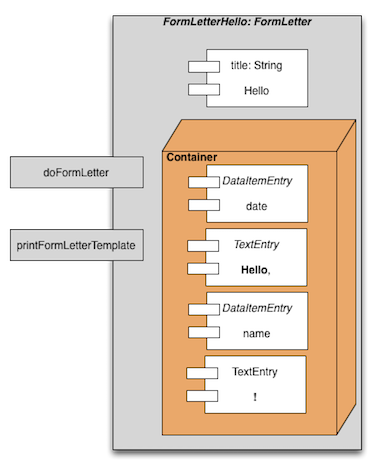
ICT-4361 Homework 5a
Purpose
This exercise will familiarize you with using
polymorphic containers and text processing in Java.
We will use the idea of “form letter
processing” to convey these ideas.
Form letters are a way of combining a form
letter template (text and placeholders for substitution) with a data
collection (say, names and addresses) and some computed variables
(such as the date) to create a useful result (say, a personalized
business letter).
As you can see from the class diagram, a
The FormLetter
is a concrete class derived from
the abstract class FormLetterTemplate
. Each instance of FormLetter
can be used to create one new form letter per Properties
object.
FormLetterTemplate
class has four public methods (other than constructors).
Two build the form letter, and two are used for producing output.
addDataItemEntry
is used to add one DataItemEntry
to the form letter
addTextEntry
is used to add one TextEntry
to the form letter
printFormLetterTemplate
outputs the form letter template itself to the provided output stream. This method
will show the placeholders.
doFormLetter
creates and outputs a form letter, using the Properties
object to provide values for all the placeholders.
In this exercise we will complete the classes for the form letter framework, and process a form letter to produce the appropriate results.
In the followup exercise for next week, the data collections and output will use files rather than internal storage.
What to Hand In
Ensure your name, and the name of the file is available in a comment at the top of
the file.
You do not need to submit files from the homework starter files that are unchanged.
Also, ensure that you have a sample of the output from the program.
If your program fails to compile, hand in your error listing as your output.
For electronic submission, “zip” your submission together into a single file, to ensure
nothing is missing;
For each question asked, provide one or two sentences summarizing your answer. Please
be both complete and succinct.
Problems
- Create and Test a Form Letter
-
Start with the homework starter files. These will give you a good framework for creating your FormLetterTemplate
. -
Examine the FormLetterEntry
abstract class, and create the two derived classesTextEntry
andDataItemEntry
. Be sure to begin to implement (or let your IDE do it) all the abstract methods in each derived class. -
Add fields and methods to the FormLetter
class. These should include a container forFormLetterEntry
s, appropriate constructors forFormLetter
, and the methods outlined in the class starter. -
Compile and run the FormLetterHello
program. When you have implemented the above, it should compile and run. -
Capture the output of your test run for your submission. -
Create a new class called MyFormLetter
, which represents another example of a form letter. It must contain at least oneTextEntry
and at least oneDataItemEntry
. This letter should be different thanFormLetterHello
, and use differentProperty
entries. Compile it, run it, and same the output of your test run for your submission. -
Optional: Run the JUnit tests and make them all pass (this might take minor changes to your classes).
You will need to ensure the JUnit libraries are on your classpath.
If you submit this optional part, be sure to capture the output showing the JUnit tests passed and include with your submission.
-
- Question: Describe why these files will not compile as they are. Be specific, and display some confirmation for your description. The answer may be stored in a text file, or incorporated as comments in your code.
Notes
-
You can put the sample classes into your NetBeans environment by putting all the files except FormLetterTest.java
into the source directory, and this remaining file into the test directory. Similar instructions for Eclipse. Or, you can also simply use ant with the providedbuild.xml
file. -
While you could create the TextEntry
andDataItemEntry
at the bottom of theFormLetterEntry.java
, please instead create them as separate, public classes. -
Note that while doing input and output in the DataItemEntry
,TextEntry
, andFormLetter
code, you should always use theout
instance passed in as a parameter, rather than codingSystem.out
into these methods. This will ensure that all your output happens properly, and jUnit tests will succeed.
In a production scenario, output fromdoFormLetter
would go to a mass mailer, or printer, or such device. -
Note that the FormLetter
class must store an ordered list ofFormLetterEntry
s. This is best handled by using one of the Collections classes in thejava.util
package. Various methods in yourFormLetter
class will iterate through the collection. For example, aList
ofFormLetterEntry
would be a reasonable collection, instantiated by, say, aLinkedList
orArrayList
ofFormLetterEntry
. Because your ordered list storesFormLetterEntry
instances, it will be able to storeTextEntry
objects andDataItemEntry
objects (through inheritance). -
Note that the Properties
object can contain things that might change for each letter (e.g., name) as well as computable things (e.g., date). -
Your addTextEntry
method creates a newTextEntry
using the provided text and adds it to your list -
Your addDataItemEntry
method creates a newDataItemEntry
using the provided name and adds it to your list -
Your doFormLetter
method should first go through each item on your list, and invoke itsdoProcess
method, providing theProperties
object it needs for substitution. Afterward, it should invokeformLetterString()
to place the result on the provided output stream. -
FormLetterHello
is the simplest test program using aFormLetter
and this set of classes, so try running it first. -
You must also be sure not to add extra spaces when printing the templates or FormLetter
, and finally when printing out the template, you must print outDataItemEntry
s as <name>. That is, a less-than, the name of the data item, and then a greater-than.
Evaluation
Criteria | Weight |
---|---|
Answer for the question, including justification | 10% |
TextEntry derived class and test | 20% |
DataItemEntry derived class and test | 20% |
FormLetter class completion | 20% |
FormLetterHello test program and output | 15% |
MyFormLetter test program and output | 15% |
JUnit tests completed and output | +10% |