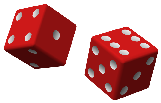
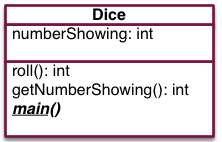
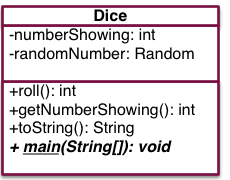
ICT-4361 Homework 2a
Purpose
What to Hand In
For this, as well as future programming exercises, hand in a listing of the program
(which must be formatted in a reasonable style) (.java file or files), and sample run(s) of the program.
Sample runs may be submitted as text files (.txt) or image files (.jpg, .png)
Remember to use our Java naming and coding conventions! Canvas submission instructions: Please pay attention to your code indentation to ensure your paper is easy to read and
understand.
Please combine multiple files into a single "zip"
archive, and save it in a location that you will remember. When you are ready to submit
the assignment, open that assignment, and submit your file as the assignment solution.
Problems
- It's Dicey
-
Create a class called Dice
to represent a single cube. The class diagram for class is shown. There may be many Dice objects, and each is responsible for remembering and reporting (on request) its current value (that is, the number showing), using its numberShowing property. The Dice object also requires a method calledroll()
that randomly selects a number from 1 to 6 for the value of the dice, and remembers it. -
Create a test main method for the Dice class that creates a Dice, and rolls it many times.
-
- Can you keep track of how many times each number (1,2,3,4,5, or 6) comes up? Describe how in a three or fewer well-written sentences, or implement it in your program.
- Does one number comes up (or is expected to come up) significantly more often than the others? If so, which one? State your hypothesis about the frequency of each number in a sentence. Back your answer up with your statistics if possible. If your program rolls a Dice object, please ensure it is rolled at least 1,000 times. You could easily roll it 1,000,000 times.
Notes
-
For the Dice class, you will need a random number generator. The way this is done in Java is to use the java.util.Random
class. After creating an instance, sayrandomNumber
, you can callrandomNumber.nextInt(6)
to return an integer, randomly distributed from zero to five. You would then add one (since a Dice objectnumberShowing
goes from one to six, not zero to five) to get the value of the roll. Where should this result be stored? -
Your main test method will have the signature public static void main(String[] args)
, as is usual, and the method should create a Dice, and invokeroll()
on it many times. -
Use a variable for the maximum number of times you want to roll()
the die, so you can see what happens as you increase the number. -
Remember: You do NOT need to keep track of each roll of the Dice object. You need only track how many times you rolled a 1, how many times you rolled a 2, how many times you rolled a 3, and so forth. -
Both a summary and detailed class diagram has been provided for this problem. For most problems, only a summary class diagram will be provided. To deepen your understanding of the connection of object-oriented analysis to object-oriented programming, please follow the class diagram for your data member names and method names. The conventions for the detailed diagram are a plus sign (+) for "public" visibility, a minus sign (-) for private visibility, and underlining for a "static" field or method. -
One method shown on the detailed class diagram is toString()
. Implementing this method will give you control of how to output aDice
object with a call likeSystem.out.println(d);
, whered
is aDice
object. Without it, you may get output likeDice@27c170f0
. If you implement it, you could get output likeDice has number 4 showing
-
For this assignment, and all subsequent assignments, please put your classes in a non-default package or packages. Be sure to ask if you can't figure out how to do this.
Evaluation
Criteria | Weight |
---|---|
Placing all Java code in a non-default package | 5% |
Creation of a Dice class with a numberShowing int data member, and its "getter". | 20% |
Creation of a Dice class object of type Random, with the variable name randomNumber, for use in the roll() method. | 20% |
Creation of a roll() member function, using the randomNumber object, which returns an appropriate int | 20% |
Keeping track of how many times each number comes up: paragraph or code | 15% |
Answering (in a comment, output, or text document) hypothesis about number frequencies. Sentence or statistics | 10% |
Captured output | 10% |