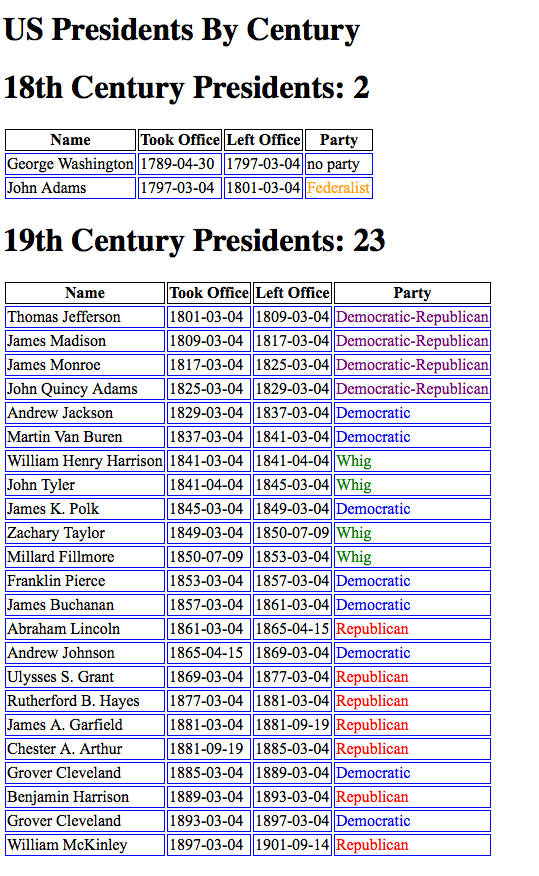
ICT-4540 Homework 6
Purpose
What to Hand In
Also, hand in screenshots from two browsers in different browser families to show
compatibility.
Problems
- Embedding the Presidents
-
Create a web page that shows presidents by century. Keep it simple! -
Load the USPresidents.xml through JavaScript. Leverage the ict4540min.js unless you are a JavaScript whiz! -
Append a div under body with an id representing the century it represents. Note that you can do this physically in the file, although it is possible to do this programmatically as well. -
For each century, include a heading of some sort that names the century, and include the count of Presidents for that century. -
Place the result for each century in the correct div. Use the document.getElementById to find the right div (or create it on the fly). -
The year the President came to office, the year the President left office, the President's party, should be displayed -
Use CSS only to style the display attractively. -
Optional: The party affiliation should color the text or background of the table cell (or row, if you prefer) for that President.
-
- Optional: Perform the same action as in Problem I, but use the JSON data source instead of the XML data source. Be sure to use the DOM's Javascript API to add the elements and attributes to the DOM.
Notes
-
You will find USPresidents.xml and presidents.css in the HWstarters ZIP file. However the version from the third assignment, where the data is integrated with PresidentBirthdays.xml, and the date transformations have already been done, is a better starting point. -
The ict4540JS.zip archive in the Week 7 material has the ICT4540 JavaScript minimal library, as well as the JSON data source. -
For the first problem, it is your choice as to how to go about selecting the correct Presidents from the XML document. You may use XSLT and apply the transformation, or you may use the JavaScript DOM API to extract the data you wish. In my opinion, the XSLT is easier. -
If you use JavaScript for problem 1, remember you are much better off creating a number of very small functions that do their job well, rather than one ball of haywire to try the whole transformation. We can discuss this further, but here is a small example to add a div programatically. - function addDiv(divName) {
- var body=document.getElementsByTagName("body")[0] ;
- var newDiv = document.createElement("div");
- var pAttr = document.createAttribute("id");
- pAttr.value=divName;
- newDiv.setAttributeNode(pAttr);
- body.appendChild(newDiv);
- }
- function addDiv(divName) {
-
When retrieving elements by getElementsByTagName, remember you are receiving an Array. If there is only one element, it is at index 0. For instance, the body tag is reasonably retrieved as document.getElementsByTagName("body")[0]
Of course it is much better to grab elements by their Id, and the corresponding getElementById method returns at most one element (it could return null if there is no such element). -
Getting the result of XPath expressions in Javascript is not too difficult, but I'd recommend a good Javascript error console and DOM inspector to do the job. Safari has a good one built in, and both Firefox and IE have nice add-ons.
If you get a blank page in your favorite browser, it might be because the browser doesn't support XML transformation, or because of the "same origin" policy we have discussed. If so, use one of the workarounds (a different browser, hosting pages in a local web server, or temporarily disabling the policy). -
The XPath API call is document.evaluate which takes 5 parameters: The XPath expression, the document you are searching (which is document for many uses, but for the loaded US Presidents document, will be the variable you loaded that into with ict4540readxml). The third and fifth parameters are usually null, and the fourth specifies the type of result you are looking for. For this problem, the most likely needed values are XPathResult.ORDERED_NODE_ITERATOR_TYPE (5) and XPathResult.NUMBER_TYPE (1). -
For a numeric result (e.g., a count()), access the numberValue property; for an iterator type, you must iterate over the results using the iterateNext() function. The default result object, if you provide only the first two parameters, is an XPathResult.ANY_TYPE, which will also work for our purposes. -
The USPresidents XML file, converted to JSON can be referenced at (by browsers that don't protect against cross-site data download) or downloaded from here: mysite.du.edu/~mschwart/ICT4540/Javascript/USPresidents.json. -
An ict4540readJson function looks just like the ict4540readxml function. There is an evaluation at the end using either JSON.parse (preferred) or eval to do the final conversion. - function ict4540readJson(url) {
- var content;
- var jsonDoc;
- var path="none";
- if (window.ActiveXObject) {
- xmlDoc = new ActiveXObject("Msxml2.FreeThreadedDOMDocument");
- xmlDoc.async = false;
- if (xmlDoc.load(url)) {
- return xmlDoc;
- }
- }
- if (window.XMLHttpRequest) {
- xmlHttp = new window.XMLHttpRequest();
- } else {
- xmlHttp = new ActiveXObject("Microsoft.XMLHTTP");
- }
- xmlHttp.open("GET", url, false);
- xmlHttp.send(null);
- content = xmlHttp.responseText;
- if ( JSON.parse )
- return JSON.parse(content);
- else
- return eval("(" + content + ")");
- }
- function ict4540readJson(url) {
Evaluation
Criteria | Weight |
---|---|
Web page | 25% |
XPath expressions | 25% |
CSS | 25% |
Output and display captures | 25% |
JSON usage | +25% |